Serialization is the process of converting a data structure or object into a sequence of bits so that it can be stored in a file or memory buffer, or transmitted across a network connection link to be reconstructed later in the same or another computer environment.
Design an algorithm to serialize and deserialize a binary tree. There is no restriction on how your serialization/deserialization algorithm should work. You just need to ensure that a binary tree can be serialized to a string and this string can be deserialized to the original tree structure.
Clarification: The input/output format is the same as how LeetCode serializes a binary tree. You do not necessarily need to follow this format, so please be creative and come up with different approaches yourself.
Example 1:
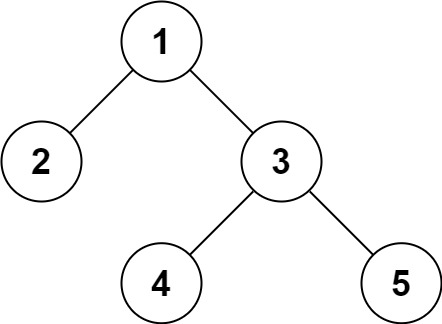
Input: root = [1,2,3,null,null,4,5] Output: [1,2,3,null,null,4,5]
Example 2:
Input: root = [] Output: []
Example 3:
Input: root = [1] Output: [1]
Example 4:
Input: root = [1,2] Output: [1,2]
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -1000 <= Node.val <= 1000
Idea: (Recursion)
We can encodes the tree to a single string. // # indicates reach to the leave Convert above tree to 12##34##56##
Time Complexity O(n)
Solution:
/**
* Definition for a binary tree node.
* function TreeNode(val) {
* this.val = val;
* this.left = this.right = null;
* }
*/
/**
* Encodes a tree to a single string.
*
* @param {TreeNode} root
* @return {string}
*/
var serialize = function(root) {
let res = [];
serializer(root, res);
return res.join(",");
};
var serializer = function(root, output) {
if (root === null) {
output.push("#");
return;
}
output.push(root.val);
serializer(root.left, output);
serializer(root.right, output);
}
// Decodes your encoded data to tree.
var deserialize = function(data) {
data = data.split(",");
let index = 0;
function deserializer(data) {
if(index > data.length || data[index] === "#") {
return null;
}
let root = new TreeNode(parseInt(data[index]));
index++;
root.left = deserializer(data);
index++;
root.right = deserializer(data);
return root;
}
return deserializer(data);
};