Given the root
of a binary tree, determine if it is a valid binary search tree (BST).
A valid BST is defined as follows:
- The left subtree of a node contains only nodes with keys less than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
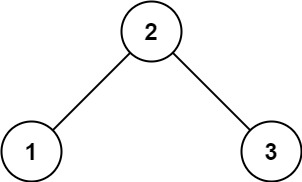
Input: root = [2,1,3] Output: true
Example 2:
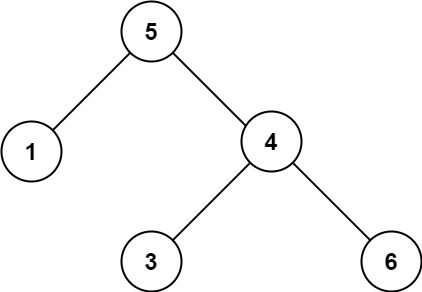
Input: root = [5,1,4,null,null,3,6] Output: false Explanation: The root node's value is 5 but its right child's value is 4.
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. -231 <= Node.val <= 231 - 1
Idea:
Use Binary Tree Inorder Traveral Template
Solution 1:
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @return {boolean}
*/
var isValidBST = function(root) {
// use inorder traversal template (2 whiles, 里左外右)
let preVal = -Infinity;
let stack = [];
while (root !== null || stack.length !== 0) {
while (root !== null) {
stack.push(root);
root = root.left;
}
root = stack.pop();
if (root.val <= preVal) return false;
preVal = root.val;
root = root.right;
}
return true;
};
Solution 2: (Recursion)
var isValidBST = function(root) {
return helper(root, null, null);
}
function helper(node, low, high) {
if (node === null) return true;
const val = node.val;
if ((low !== null && val <= low) || (high !== null && val >= high))
return false;
return helper(node.right, val, high) && helper(node.left, low, val);
}