Given the head
of a linked list, remove the nth
node from the end of the list and return its head.
Follow up: Could you do this in one pass?
Example 1:
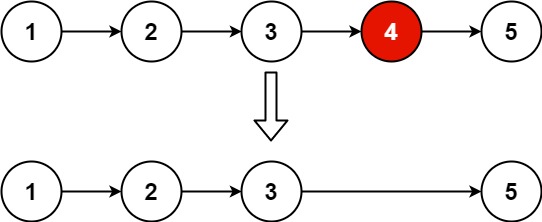
Input: head = [1,2,3,4,5], n = 2 Output: [1,2,3,5]
Example 2:
Input: head = [1], n = 1 Output: []
Example 3:
Input: head = [1,2], n = 1 Output: [1]
Constraints:
- The number of nodes in the list is
sz
. 1 <= sz <= 30
0 <= Node.val <= 100
1 <= n <= sz
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} head
* @param {number} n
* @return {ListNode}
*/
var removeNthFromEnd = function(head, n) {
if (head.next === null)
return null;
let first = new ListNode();
first.next = head;
let second = first;
// first point to n + 1
first = first.next;
while (n > 0) {
first = first.next;
n--;
}
// first already reach to the end null, means need to remove the first node
if (first === null) {
head = head.next;
} else {
// maintaining N nodes apart between first and second pointer
while (first !== null) {
first = first.next;
second = second.next;
}
// Now second pointer to the node that need to be removed
// remove that node now
second.next = second.next.next;
}
return head;
};